The paceval. application programming interface
The API is simple to use and very powerful. It is written in standard C/C++, but you can easily create your own interfaces for your development environment and your programming language.
For C/C++ just
① add paceval_main.cpp to your project and
② add the paceval.-library for your environment to your application directory (.dll or .so) or to your project (.lib or .a), open screenshots below.For other programming languages you can use the Foreign function interface (FFI).
For the cloud-solution, please, follow this link to our documentation at paceval-service | paceval | SwaggerHub.
If you have a paceval. source code license, you can easily customize and extend the API to meet your needs. Or create new solutions for your own target hardware.
APPLICATION PROGRAMMING INTERFACE – paceval.
mathematical engine, Version 4.24
Release: September 2023, https://github.com/paceval
(see paceval sources (external) documentation)
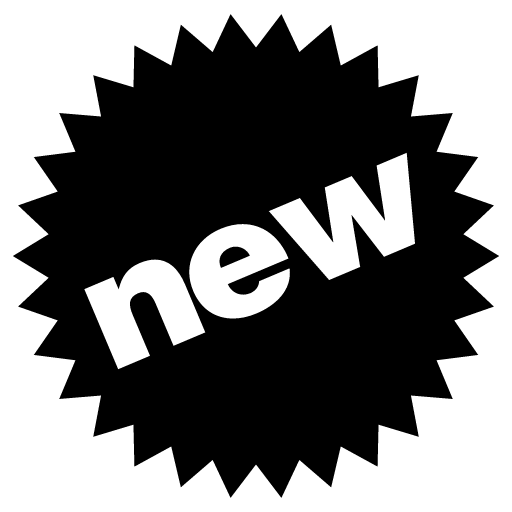
OVERVIEW
- paceval_CreateComputation(…) – Creates a paceval.-Computation object.
- paceval_CreateMultipleComputations(…) – Creates multiple paceval.-Computation objects in one step.
- paceval_ExistComputation(…) – Determines whether a particular paceval.-Computation object has been created.
- paceval_DeleteComputation(…) – Deletes a paceval.-Computation object.
- paceval_dGetComputationResult(…) – Performs a calculation with a set of values.
- paceval_GetIsError(…) – Determines whether an error has occurred.
- paceval_GetErrorInformation(…) – Returns the error code value.
- paceval_GetComputationVersionString(…) – Returns the version string.
- paceval_InitializeLibrary(…) – Initializes the paceval.-library.
- paceval_FreeLibrary(…) – Frees the paceval.-library.
- paceval_dConvertFloatToString(…) – Converts a floating point number to a string.
- paceval_dConvertStringToFloat(…) – Converts a to a string to a floating point number.
- paceval_dGetComputationResultExt(…) – Performs multiple calculations in parallel on a single paceval.-Computation object with multiple sets of values.
- paceval_dGetMultipleComputationsResults(…) – Performs multiple calculations in parallel on multiple paceval.-Computation objects with the same set of values.
- paceval_dGetMultipleComputationsResultsExt(…) – Performs multiple calculations in parallel on multiple paceval.-Computation objects with multiple sets of values.
- paceval_dmathv(…) – A convenience function to get results of computations in a single step.
- paceval_dmathvi(…) – A convenience function to get results of computations in a single step with Trusted Interval Computation.
- paceval_CreateXMLFromParameters(…) – A helper function to create XML data representing a paceval.-Computation object.
- paceval_ReadParametersFromXML(…) – A helper function to read XML data representing a paceval.-Computation object.
- paceval_GetComputationInformationXML(…) – A helper function for getting data from a paceval.-Computation object
- paceval_CreateErrorInformationText(…) – A helper function to create error information in text from a paceval.-Computation object.
- paceval_GetErrorTypeMessage(…) – A helper function to create an error message in text from an error type.
- paceval_SetCallbackUserFunction(…) – Allows the definition of custom user functions.
- How to easily call paceval.-library functions from another programming language – Background and examples : Foreign function interface (FFI)
PACEVAL_HANDLE paceval_CreateComputation(
const char* functionString_in,
unsigned long numberOfVariables_in,
const char* variables_in,
bool useInterval_in,
paceval_callbackStatusType* paceval_callbackStatus_in);
NAME
paceval_CreateComputation
DESCRIPTION
Creates a paceval.-Computation object for a mathematical function and its variables, i.e. in mathematical notation
You can also set whether calculations should be carried out with or without interval arithmetic.
Annotation: The reference HANDLE returned when calling paceval_CreateComputation() is a token that uniquely represents the paceval.-Computation object managed by the paceval.-library. This token can then be used to carry out calculations and queries on the paceval.-Computation object without having to re-pass the possibly very long function.
PARAMETERS
const char* functionString_in – Points to a null-terminated string to be used as the function to work with.
see also Product brief-Supported terms
unsigned long numberOfVariables_in – Specifies the number of variables to work with. E.g. if the variables are “xValue yValue zValue” or “x y z” numberOfVariables_in must be set to 3.
const char* variables_in – Points to a null-terminated string specifying the names of the working variables. Generally the variables must be separated by a blank. E.g. the variables xValue, yValue, zValue must be set with “xValue yValue zValue” or the variables x, y, z must be set with “x y z”.
bool useInterval_in – Enables or disables the Trusted Interval Computation, TINC (paceval. specific) putting bounds on rounding errors and measurement errors of the computation system to yield reliable results.
paceval_callbackStatusType* paceval_callbackStatus_in – A user-defined callback function to give status information of the paceval.-Computation object, see paceval_main.h:
typedef bool paceval_callbackStatusType(const PACEVAL_HANDLE, const paceval_eStatusTypes, const int);
RETURN VALUE
PACEVAL_HANDLE – The return value is the HANDLE of the created paceval-Computation object. paceval_GetIsError() should be called to check whether an error has occurred. To get more information about the error, call paceval_GetErrorInformation(). This object can be used (e.g. with paceval_dGetComputationResult(), paceval_dGetMultipleComputationsResults(), paceval_dmathv() or paceval_dmathvi()) and must be deleted with paceval_DeleteComputation().
FOREIGN FUNCTION INTERFACE
void* pacevalLibrary_CreateComputation(const char* functionString_in,
unsigned long numberOfVariables_in, const char* variables_in,
bool useInterval_in, void* paceval_callbackStatus_in);
Please, read Foreign function interface for more information and examples.
EXAMPLE
see USING paceval. – A SIMPLE CODE EXAMPLE
see demo application AppCalculation “main.cpp” of the paceval. Software Development Kit
see examples “paceval_example1.cpp”, “paceval_example3.cpp”, “paceval_example4.cpp”, “paceval_example5.cpp” or “paceval_example6.cpp” of the paceval. Software Development Kit
EXAMPLE C/C++
//This code creates a mathematical function and then also performs a calculation without interval arithmetic.
//For an example with interval arithmetic, just look here: https://paceval.com/simple-code-example/
PACEVAL_HANDLE handle_pacevalComputation_Addition = NULL;
double result_Addition;
double valuesVariablesArray_Addition[1];
int errType;
handle_pacevalComputation_Addition = paceval_CreateComputation(“5+x”, 1, “x”, false, NULL);
valuesVariablesArray_Addition[0] = 2.6; //value for variable x
result_Addition = paceval_dGetComputationResult(handle_pacevalComputation_Addition, valuesVariablesArray_Addition, NULL, NULL);
paceval_DeleteComputation(handle_pacevalComputation_Addition);
//result_Addition will be 7.6 (5+2.6)
bool paceval_CreateMultipleComputations(
PACEVAL_HANDLE handle_pacevalComputations_out[],
const char* functionStrings_in[],
unsigned long numberOfpacevalComputations_in,
unsigned long numberOfVariables_in,
const char* variables_in,
bool useInterval_in,
paceval_callbackStatusType* paceval_callbackStatus_in);
NAME
paceval_CreateMultipleComputations
DESCRIPTION
Creates paceval.-Computation objects for multiple mathematical functions and one set of variables in a single step, i.e. in mathematical notation
This is useful for a set of functions with the same number of variables and the same names for the variables (e.g. for Artificial neural network functions or Decison trees).
Annotation: paceval_CreateMultipleComputations() is also very useful in a Kubernetes cluster environment. It simplifies the creation and usage of all sets of paceval.-Computation objects on the same worker node (e.g. with paceval_dGetMultipleComputationsResults()).
PARAMETERS
PACEVAL_HANDLE handle_pacevalComputations_out[] – If paceval_CreateMultipleComputations() is succesful this retrieves the array of HANDLE of the created paceval-Computation objects.
const char* functionStrings_in[] – Points to an array of null-terminated strings to be used as the functions to work with for the created paceval-Computation objects.
see also Product brief-Supported terms
unsigned long numberOfpacevalComputations_in – Specifies the number of paceval-Computation objects to create. E.g. if the functions to work with are “sin(x)” and “cos(x)” numberOfpacevalComputations_in must be set to 2.
unsigned long numberOfVariables_in – Specifies the number of variables to work with. E.g. if the variables are “xValue yValue zValue” or “x y z” numberOfVariables_in must be set to 3.
const char* variables_in – Points to a null-terminated string specifying the names of the working variables. Generally the variables must be separated by a blank. E.g. the variables xValue, yValue, zValue must be set with “xValue yValue zValue” or the variables x, y, z must be set with “x y z”.
bool useInterval_in – Enables or disables the Trusted Interval Computation, TINC (paceval. specific) putting bounds on rounding errors and measurement errors of the computation system to yield reliable results.
paceval_callbackStatusType* paceval_callbackStatus_in – A user-defined callback function to give status information of the paceval.-Computation objects, see paceval_main.h:
typedef bool paceval_callbackStatusType(const PACEVAL_HANDLE, const paceval_eStatusTypes, const int);
RETURN VALUE
bool – If the function succeeds, the return value is true and handle_pacevalComputations_out[] contains the paceval-Computation objects. Each of these objects can be used individually (e.g. with paceval_dGetComputationResult(), paceval_dGetMultipleComputationsResults(), paceval_dmathv() or paceval_dmathvi()) and must be deleted with paceval_DeleteComputation().
FOREIGN FUNCTION INTERFACE
bool pacevalLibrary_CreateMultipleComputations(void* handle_pacevalComputations_out[],
const char* functionStrings_in[], unsigned long numberOfpacevalComputations_in,
unsigned long numberOfVariables_in, const char* variables_in, bool useInterval_in,
void* paceval_callbackStatus_in);
Please, read Foreign function interface for more information and examples.
EXAMPLE
see example “paceval_example6.cpp” of the paceval. Software Development Kit
//This code creates two mathematical functions in parallel and then performs a calculation on the first function
//without interval arithmetic.
//Although the second mathematical function is not used, you can easily extend the example to do so.
//For an example with interval arithmetic, just look here: https://paceval.com/simple-code-example/
PACEVAL_HANDLE* handle_pacevalComputationsArray = new PACEVAL_HANDLE[2];
const char** functionStringsArray = new const char*[2];
char* functionString_Addition = new char[sizeof(“5+x”) + 1];
char* functionString_Multiplication = new char[sizeof(“y*7.8”) + 1];
double result_Addition;
double valuesVariablesArray_Addition[1];
bool hasError;
strcpy(functionString_Addition, “5+x”);
strcpy(functionString_Multiplication, “y*7.8”);
functionStringsArray[0] = functionString_Addition;
functionStringsArray[1] = functionString_Multiplication;
hasError = paceval_CreateMultipleComputations(handle_pacevalComputationsArray, functionStringsArray, 2, 2, “x y”, false, NULL);
delete[] functionString_Addition;
delete[] functionString_Multiplication;
delete[] functionStringsArray;
valuesVariablesArray_Addition[0] = 2.6; //value for variable x
result_Addition = paceval_dGetComputationResult(handle_pacevalComputationsArray[0], valuesVariablesArray_Addition, NULL, NULL);
//result_Addition will be 7.6 (5+2.6)
paceval_DeleteComputation(handle_pacevalComputationsArray[0]);
paceval_DeleteComputation(handle_pacevalComputationsArray[1]);
delete[] handle_pacevalComputationsArray;
bool paceval_ExistComputation(
PACEVAL_HANDLE handle_pacevalComputation_in);
NAME
paceval_ExistComputation
DESCRIPTION
Determines whether a particular paceval.-Computation object has been created.
PARAMETERS
PACEVAL_HANDLE handle_pacevalComputation_in – Identifies the paceval.-Computation object to be questioned.
RETURN VALUE
bool – If the object exists, the return value is true. If the specified HANDLE is invalid or the object does not exist, the return value is false.
FOREIGN FUNCTION INTERFACE
bool paceval_ExistComputation(void* handle_pacevalComputation_in);
Please, read Foreign function interface for more information and examples.
bool paceval_DeleteComputation(
PACEVAL_HANDLE handle_pacevalComputation_in);
NAME
paceval_DeleteComputation
DESCRIPTION
Deletes a paceval.-Computation object.
PARAMETERS
PACEVAL_HANDLE handle_pacevalComputation_in – Identifies the paceval.-Computation object to delete.
RETURN VALUE
bool – If the function succeeds, the return value is true. If the given HANDLE is not valid the return value is false.
FOREIGN FUNCTION INTERFACE
bool pacevalLibrary_DeleteComputation(void* handle_pacevalComputation_in);
Please, read Foreign function interface for more information and examples.
EXAMPLE
see demo application AppCalculation “main.cpp” of the paceval. Software Development Kit
see examples “paceval_example1.cpp”, “paceval_example3.cpp”, “paceval_example4.cpp”, “paceval_example5.cpp” or “paceval_example6.cpp” of the paceval. Software Development Kit
double paceval_dGetComputationResult(
PACEVAL_HANDLE handle_pacevalComputation_in,
double values_in[],
double* trustedMinResult_out,
double* trustedMaxResult_out);
use paceval_fGetComputationResult(…) for float values
use paceval_ldGetComputationResult(…) for long double values
NAME
paceval_dGetComputationResult
paceval_fGetComputationResult
paceval_ldGetComputationResult
DESCRIPTION
Performs a calculation on a paceval.-Computation object with the variables declared by paceval_CreateComputation() and with a set of values for those variables, i.e. in mathematical notation
PARAMETERS
PACEVAL_HANDLE handle_pacevalComputation_in – Identifies the paceval.-Computation object.
double values_in[] – Array of double defining the values of the variables.
double* trustedMinResult_out – If Trusted Interval Computation was enabled by paceval_CreateComputation() this retrieves the minimum/lower interval limit of the computation.
Annotation: In case of an error the value is not specified.
double* trustedMaxResult_out – If Trusted Interval Computation was enabled by paceval_CreateComputation() this retrieves the maximum/upper interval limit of the computation.
Annotation: In case of an error the value is not specified.
RETURN VALUE
double – If the function succeeds, the return value is the result of the computation.
paceval_GetIsError() should be called to check whether an error has occurred. To get more information about the error, call paceval_GetErrorInformation().
Annotation: In case of an error the return value is not specified.
FOREIGN FUNCTION INTERFACE
double pacevalLibrary_dGetComputationResult(void* handle_pacevalComputation_in,
double values_in[], double* trustedMinResult_out, double* trustedMaxResult_out);
float pacevalLibrary_fGetComputationResult(void* handle_pacevalComputation_in,
float values_in[], float* trustedMinResult_out, float* trustedMaxResult_out);
long double pacevalLibrary_ldGetComputationResult(void* handle_pacevalComputation_in,
long double values_in[], long double* trustedMinResult_out, long double* trustedMaxResult_out);
Please, read Foreign function interface for more information and examples.
EXAMPLE
see USING paceval. – A SIMPLE CODE EXAMPLE
see demo application AppCalculation “main.cpp” of the paceval. Software Development Kit
see example “paceval_example1.cpp” of the paceval. Software Development Kit
EXAMPLE C/C++
//This code creates a mathematical function and then also performs a calculation without interval arithmetic.
//For an example with interval arithmetic, just look here: https://paceval.com/simple-code-example/
PACEVAL_HANDLE handle_pacevalComputation_Addition = NULL;
double result_Addition;
double valuesVariablesArray_Addition[1];
int errType;
handle_pacevalComputation_Addition = paceval_CreateComputation(“5+x”, 1, “x”, false, NULL);
valuesVariablesArray_Addition[0] = 2.6; //value for variable x
result_Addition = paceval_dGetComputationResult(handle_pacevalComputation_Addition, valuesVariablesArray_Addition, NULL, NULL);
paceval_DeleteComputation(handle_pacevalComputation_Addition);
//result_Addition will be 7.6 (5+2.6)
bool paceval_GetIsError(
PACEVAL_HANDLE handle_pacevalComputation_in);
NAME
paceval_GetIsError
DESCRIPTION
Determines whether an error has occurred. To get more information about the error, call paceval_GetErrorInformation().
PARAMETERS
PACEVAL_HANDLE handle_pacevalComputation_in – Identifies the paceval.-Computation object.
RETURN VALUE
bool – If an error has occurred, the return value is true. Otherwise it is false.
FOREIGN FUNCTION INTERFACE
bool pacevalLibrary_GetIsError(void* handle_pacevalComputation_in);
Please, read Foreign function interface for more information and examples.
EXAMPLE
see demo application AppCalculation “main.cpp” of the paceval. Software Development Kit
see examples “paceval_example1.cpp”, “paceval_example5.cpp” or “paceval_example6.cpp” of the paceval. Software Development Kit
int paceval_GetErrorInformation(
PACEVAL_HANDLE handle_pacevalComputation_in,
char* lastError_strOperator_out,
long* lastError_errPosition_out);
NAME
paceval_GetErrorInformation
DESCRIPTION
The paceval_GetErrorInformation() function returns the paceval.-Computation object error type value.
Possible errors are analysis and computation errors. Errors during the analysis should be checked after creation of the object like paceval_CreateComputation().
Errors during the computation should be checked after paceval_GetComputationResult().
PARAMETERS
PACEVAL_HANDLE handle_pacevalComputation_in – Identifies the paceval.-Computation object.
char* lastError_strOperator_out – Buffer to get the operator causing the error.
Annotation: Maximum size of the retrieved null-terminated string is 255 per “#define PACEVAL_MAXERR 255”, see paceval_main.h.
long* lastError_errPosition_out – Position in the function where the error occurred.
RETURN VALUE
int – The return value is the paceval.-Computation object error, see also paceval_eErrorTypes in paceval_main.h and below. You can retrieve further information for the error type via paceval_CreateErrorInformationText(), e.g. to present this to the user.
PACEVAL_ERR_NO_ERROR = 0: No error occured for this computation object.
PACEVAL_ERR_FATAL_INITIALIZE_LIBRARY_NOT_CALLED = 10: Initialization of paceval. with paceval_InitializeLibrary() is missing.
PACEVAL_ERR_FATAL_NO_LICENSE = 11: Get in contact with us via info@paceval.com for a license.
PACEVAL_ERR_FATAL_CONNECTING_SERVER = 15: This is a placeholder for the error codes when using an external library, e.g. like curl.
PACEVAL_ERR_FATAL_PARAMETERS_SERVER = 16: This is a placeholder for the error codes when using an external library, e.g. like curl.
PACEVAL_ERR_ANALYSIS_UNKNOWN_SIGN_OR_FUNCTION = 110: The function string contains an unknown sign or subfunction.
PACEVAL_ERR_ANALYSIS_BRACKETS = 111: Incorrect brackets are set in the function string.
PACEVAL_ERR_ANALYSIS_UNKNOWN_CONSTANT_OR_VARIABLE = 112: The function string contains an unknown constant factor or variable.
PACEVAL_ERR_ANALYSIS_COMMENT = 113: An invalid comment in the function string.
PACEVAL_ERR_ANALYSIS_NUMBER_OF_VARIABLES = 114: The specified number of variables is not the number of the declared variables.
PACEVAL_ERR_ANALYSIS_MISPLACED_SIGN_CALCULATION = 115: An operator is set incorrectly in the function string.
PACEVAL_ERR_ANALYSIS_UNEXPECTED_END = 116: An unexpected end in the function string.
PACEVAL_ERR_ANALYSIS_WRONGLY_USED_FUNCTION = 117: A subfunction is set incorrectly in the function string.
PACEVAL_ERR_ANALYSIS_NO_USER_FUNCTION = 118: No user function and no operations.
PACEVAL_ERR_ANALYSIS_UNKNOWN_CHARACTER = 119: The function string contains an unknown character.
PACEVAL_ERR_ANALYSIS_WRONGLY_USED_CONSTANT_OR_VALUE = 120: A constant factor or value is not correctly used.
PACEVAL_ERR_ANALYSIS_MISSING_OPERATOR = 121: An operator is missing for operands.
PACEVAL_ERR_ANALYSIS_OUT_OF_MEMORY = 122: Analysis out of memory.
PACEVAL_ERR_ANALYSIS_UNKNOWN_OBJECT_TYPE = 124: An invalid object-type was passed.
PACEVAL_ERR_ANALYSIS_MEMORY_CLEANUP = 126: An error has occured in the memory cleanup.
PACEVAL_ERR_ANALYSIS_USER_ABORT = 127: The analysis was aborted by the user.
PACEVAL_ERR_ANALYSIS_MATH_OS_NOT_SUPPORTED = 128: The operating system may not be supported due to known mathematical errors.
PACEVAL_ERR_ANALYSIS_NO_COMMUNITY_FEATURE = 130: This feature is not supported in the free version of paceval.
PACEVAL_ERR_COMPUTATION_HANDLE_INVALID = 140: An invalid handle was passed.
PACEVAL_ERR_COMPUTATION_MULTIPLICATION = 141: A multiplication causes an error.
PACEVAL_ERR_COMPUTATION_DIVISION = 142: A division causes an error.
PACEVAL_ERR_COMPUTATION_FUNCTION = 143: The result of the calculation is not valid.
PACEVAL_ERR_COMPUTATION_ADDITION = 144: An addition causes an error.
PACEVAL_ERR_COMPUTATION_SUBTRACTION = 145: A subtraction causes an error.
PACEVAL_ERR_COMPUTATION_UNSPECIFIED = 150: A not specified user-function causes an error.
PACEVAL_ERR_COMPUTATION_INTERVAL_RESULT = 151: The interval result of the calculation is not trusted.
PACEVAL_ERR_COMPUTATION_USER_ABORT = 152: The calculation was aborted by the user.
PACEVAL_ERR_COMPUTATION_RESULT = 153: The result of the calculation is not trusted.
PACEVAL_ERR_COMPUTATION_BUSY = 154: The referenced computation object is busy.
PACEVAL_ERR_COMPUTATION_USER_COMPILER_NOT_SUPPORTS_LONG_DOUBLE = 160: Your compiler does not support long double floating-point data type.
FOREIGN FUNCTION INTERFACE
int pacevalLibrary_GetErrorInformation(void* handle_pacevalComputation_in,
char* lastError_strOperator_out, long* lastError_ePosition_out);
Please, read Foreign function interface for more information and examples.
EXAMPLE
see demo application AppCalculation “main.cpp” of the paceval. Software Development Kit
int paceval_GetComputationVersionString(
PACEVAL_HANDLE handle_pacevalComputation_in,
char* paceval_strVersion_out);
NAME
paceval_GetComputationVersionString
DESCRIPTION
Returns the version string including the version number of the paceval.-Computation object.
PARAMETERS
PACEVAL_HANDLE handle_pacevalComputation_in – Identifies the paceval.-Computation object.
char* paceval_strVersion_out – Points to the buffer that will receive the string. Set paceval_strVersion_in to NULL to receive the length of the version string, see RETURN VALUE.
RETURN VALUE
int – The return value is the length of the version string.
FOREIGN FUNCTION INTERFACE
int pacevalLibrary_GetComputationVersionString(void* handle_pacevalComputation_in,
char* paceval_strVersion_out);
Please, read Foreign function interface for more information and examples.
EXAMPLE
see demo application AppCalculation “main.cpp” of the paceval. Software Development Kit
see examples “paceval_example1.cpp”, “paceval_example5.cpp” or “paceval_example6.cpp” of the paceval. Software Development Kit
paceval_InitializeLibrary(
const char* initString_in);
NAME
paceval_InitializeLibrary
DESCRIPTION
You must call paceval_InitializeLibrary() before you use paceval.-API function calls in your code.
Annotation: In case you use the standard Windows library version this loads and initializes the paceval_win32.dll for 32bit Windows-Applications (x86) or paceval_win64i.dll for 64bit Windows-Applications (x64).
PARAMETERS
const char* initString_in – This parameter is not used in the non-commercial version. Just pass NULL pointer (e.g. “paceval_InitializeLibrary(NULL);”).
RETURN VALUE
bool – If the function succeeds, the return value is true. Otherwise it is false.
FOREIGN FUNCTION INTERFACE
bool pacevalLibrary_Initialize(const char* initString_in);
Please, read Foreign function interface for more information and examples.
EXAMPLE
see demo application AppCalculation “main.cpp” of the paceval. Software Development Kit
see examples “paceval_example1.cpp”, “paceval_example2.cpp”, “paceval_example3.cpp”, “paceval_example4.cpp”, “paceval_example5.cpp” or “paceval_example6.cpp” of the paceval. Software Development Kit
paceval_FreeLibrary();
NAME
paceval_FreeLibrary
DESCRIPTION
You must call paceval_FreeLibrary() at the end of your application.
Annotation: In case you use the standard Windows library version this frees the paceval_win32.dll for 32bit Windows-Applications (x86) or paceval_win64i.dll for 64bit Windows-Applications (x64).
RETURN VALUE
bool – If the function succeeds, the return value is true. Otherwise it is false.
FOREIGN FUNCTION INTERFACE
bool pacevalLibrary_Free();
Please, read Foreign function interface for more information and examples.
EXAMPLE
see demo application AppCalculation “main.cpp” of the paceval. Software Development Kit
see examples “paceval_example1.cpp”, “paceval_example2.cpp”, “paceval_example3.cpp”, “paceval_example4.cpp”, “paceval_example5.cpp” or “paceval_example6.cpp” of the paceval. Software Development Kit
int paceval_dConvertFloatToString(
char* destinationString_in,
double float_in);
use paceval_fConvertFloatToString(…) for float values
use paceval_ldConvertFloatToString(…) for long double values
NAME
paceval_dConvertFloatToString
paceval_fConvertFloatToString
paceval_ldConvertFloatToString
DESCRIPTION
Converts a floating point number to a string.
PARAMETERS
char* destinationString_in – Points to the buffer that will receive the string of the coverted floating point number.
Annotation: In case of an error the buffer is not specified.
double float_in – The floating point number to convert.
RETURN VALUE
int – If the function succeeds, the return value is >0 and gives the precision of the conversion. Otherwise the return value is <0.
FOREIGN FUNCTION INTERFACE
int pacevalLibrary_dConvertFloatToString(char* destinationString_in,
double float_in);
int pacevalLibrary_fConvertFloatToString(char* destinationString_in,
float float_in);
int pacevalLibrary_ldConvertFloatToString(char* destinationString_in,
long double float_in);
Please, read Foreign function interface for more information and examples.
EXAMPLE
see demo application AppCalculation “main.cpp” or “graph.cpp” of the paceval. Software Development Kit
see examples “paceval_example1.cpp”, “paceval_example2.cpp”, “paceval_example3.cpp”, “paceval_example4.cpp” or “paceval_example6.cpp” of the paceval. Software Development Kit
double paceval_dConvertStringToFloat(
const char* sourceString_in,
int* errorType_out,
long* errPosition_out,
bool useInterval_in,
double* trustedMinResult_out,
double* trustedMaxResult_out);
use paceval_fConvertStringToFloat(…) for float values
use paceval_ldConvertStringToFloat(…) for long double values
NAME
paceval_dConvertStringToFloat
paceval_fConvertStringToFloat
paceval_ldConvertStringToFloat
DESCRIPTION
Converts a to a string to a floating point number. In case a caluclation is given in the string the result is converted.
PARAMETERS
const char* sourceString_in – The string to convert.
int* errorType_out – Points to the buffer that will receive the paceval.-Computation object error, see also paceval_eErrorTypes in paceval_main.h or paceval_GetErrorInformation().
long* errPosition_out – Position in the string where the error occurred.
bool useInterval_in – Enables or disables the Trusted Interval Computation, TINC (paceval. specific) for the conversion.
double* trustedMinResult_out – If Trusted Interval Computation was enabled this retrieves the minimum/lower interval limit of the conversion.
Annotation: In case of an error the value is not specified.
double* trustedMaxResult_out – If Trusted Interval Computation was enabled this retrieves the maximum/upper interval limit of the conversion.
Annotation: In case of an error the value is not specified.
RETURN VALUE
double – If the function succeeds, the return value is the result of the conversion.
Annotation: In case of an error the return value is not specified.
FOREIGN FUNCTION INTERFACE
double pacevalLibrary_dConvertStringToFloat(const char* sourceString_in,
int* errorType_out, long* errPosition, bool useInterval_in,
double* trustedMinResult_out, double* trustedMaxResult_out);
float pacevalLibrary_fConvertStringToFloat(const char* sourceString_in,
int* errorType_out, long* errPosition, bool useInterval_in,
float* trustedMinResult_out, float* trustedMaxResult_out);
long double pacevalLibrary_ldConvertStringToFloat(const char* sourceString_in,
int* errorType_out, long* errPosition, bool useInterval_in,
long double* trustedMinResult_out, long double* trustedMaxResult_out);
Please, read Foreign function interface for more information and examples.
EXAMPLE
see demo application AppCalculation “main.cpp” or “graph.cpp” of the paceval. Software Development Kit
see examples “paceval_example1.cpp” or “paceval_example6.cpp” of the paceval. Software Development Kit
bool paceval_dGetComputationResultExt(
PACEVAL_HANDLE handle_pacevalComputation_in,
double values_in[],
unsigned long numberOfCalculations_in,
double* results_out,
double* trustedMinResults_out,
double* trustedMaxResults_out,
int* errorTypes_out);
use paceval_fGetComputationResultExt(…) for float values
use paceval_ldGetComputationResultExt(…) for long double values
NAME
paceval_dGetComputationResultExt
paceval_fGetComputationResultExt
paceval_ldGetComputationResultExt
DESCRIPTION
Performs multiple calculations in parallel on a single paceval.-Computation object with the variables declared by paceval_CreateComputation() and with multiple sets of values for those variables, i.e. in mathematical notation
You should use paceval_dGetComputationResultExt() to get many results for a single computaton at once. This is useful for example when you plot 2D- or 3D-functions and graphics very fast like in the screenshots.
PARAMETERS
PACEVAL_HANDLE handle_pacevalComputation_in – Identifies the paceval.-Computation object.
double values_in[] – Array of double defining the multiple values of the variables.
unsigned long numberOfCalculations_in – Specifies the number of different calculations you want to run. This number specifies the size of the array values_in[]. E.g. if the number of variables declared is 50 and the number of calculations is 1000 the size of the array values_in[] must be 50*1000. The order of the array values_in[] must be variable values for the first calculation, then variable values for the second calculation and so on.
double* results_out – Pointer to an array for the results of the computation with the multiple values of the variables.
Annotation: In case of an error the values are not specified.
double* trustedMinResult_out – Pointer to an array for the minimum/lower interval limits.
Annotation: In case of an error the values are not specified.
double* trustedMaxResult_out– Pointer to an array for the maximum/upper interval limits.
Annotation: In case of an error the values are not specified.
int* errorTypes_out – Pointer to an array of the paceval.-Computation object errors, see also paceval_eErrorTypes in paceval_main.h or paceval_GetErrorInformation().
RETURN VALUE
bool – The return value indicates paceval.-Computation object errors. You should check the array errorTypes_out in this case.
FOREIGN FUNCTION INTERFACE
bool pacevalLibrary_dGetComputationResultExt(void* handle_pacevalComputation_in,
double values_in[], unsigned long numberOfCalculations_in, double* results_out,
double* trustedMinResults_out, double* trustedMaxResults_out,
int* errorTypes_out);
bool pacevalLibrary_fGetComputationResultExt(void* handle_pacevalComputation_in,
float values_in[], unsigned long numberOfCalculations_in, float* results_out,
float* trustedMinResults_out, float* trustedMaxResults_out,
int* errorTypes_out);
bool pacevalLibrary_ldGetComputationResultExt(void* handle_pacevalComputation_in,
long double values_in[], unsigned long numberOfCalculations_in, long double* results_out,
long double* trustedMinResults_out, long double* trustedMaxResults_out,
int* errorTypes_out);
Please, read Foreign function interface for more information and examples.
EXAMPLE
see demo application AppCalculation “graph.cpp” of the paceval. Software Development Kit
see example “paceval_example3.cpp” of the paceval. Software Development Kit
//This code creates a mathematical function with two variables and then performs calculations in parallel
//with three different sets of values for those variables.
//For an example with interval arithmetic, just look here: https://paceval.com/simple-code-example/
double* results = new double[3];
double valuesVariablesArray_Addition[6];
int* errorTypes = new int[3];
bool hasError;
handle_pacevalComputation_Addition = paceval_CreateComputation(“5+x*y”, 2, “x y”, false, NULL);
valuesVariablesArray_Addition[0] = 2.6; //first value for variable x
valuesVariablesArray_Addition[1] = 5.8; //first value for variable y
valuesVariablesArray_Addition[2] = -1.2; //second value for variable x
valuesVariablesArray_Addition[3] = 6.9; //second value for variable y
valuesVariablesArray_Addition[4] = -1.8; //third value for variable x
valuesVariablesArray_Addition[5] = 12.1; //third value for variable y
hasError = paceval_dGetComputationResultExt(handle_pacevalComputation_Addition, valuesVariablesArray_Addition, 3, &results[0], NULL, NULL, &errorTypes[0]);
//results[0] will be 20.08 (5+2.6*5.8)
//results[1] will be -3.28 (5-1.2*6.9)
//results[2] will be -16.78 (5-1.8*12.1)
paceval_DeleteComputation(handle_pacevalComputation_Addition);
delete[] errorTypes;
delete[] results;
bool paceval_dGetMultipleComputationsResults(
PACEVAL_HANDLE handle_pacevalComputations_in[],
unsigned long numberOfpacevalComputations_in,
double values_in[],
double* results_out,
double* trustedMinResults_out,
double* trustedMaxResults_out,
int* errorTypes_out);
use paceval_fGetMultipleComputationsResults(…) for float values
use paceval_ldGetMultipleComputationsResults(…) for long double values
NAME
paceval_dGetMultipleComputationsResults
paceval_fGetMultipleComputationsResults
paceval_ldGetMultipleComputationsResults
DESCRIPTION
Performs multiple calculations in parallel on multiple paceval.-Computation objects using the variables declared by paceval_CreateComputation() and with the same set of values for these variables, i.e. in mathematical notation
You should use paceval_dGetMultipleComputationsResults() to get results for multiple computations at once (e.g. for Artificial neural network functions or Decision trees).
Annotation: You must use the same number of variables and the same declared variables. To do so you can simply declare all variables in each of the the calls for paceval_CreateComputation(). There is no performance issue in case a variable is declared but not used by a paceval.-Computation object.
PARAMETERS
PACEVAL_HANDLE handle_pacevalComputation_in[] – Array of the paceval.-Computation objects.
unsigned long numberOfpacevalComputations_in – Specifies the number of the paceval.-Computation objects.
double values_in[] – Array of double defining the values of the variables.
double* results_out – Pointer to an array for the results of the multiple computations.
Annotation: In case of an error the values are not specified.
double* trustedMinResult_out – Pointer to an array for the minimum/lower interval limits.
Annotation: In case of an error the values are not specified.
double* trustedMaxResult_out – Pointer to an array for the maximum/upper interval limits.
Annotation: In case of an error the value is not specified.
int* errorTypes_out – Pointer to an array of the paceval.-Computation object errors, see also paceval_eErrorTypes in paceval_main.h or paceval_GetErrorInformation().
RETURN VALUE
bool – The return value indicates paceval.-Computation object errors. You should check the array errorTypes_out in this case.
FOREIGN FUNCTION INTERFACE
bool pacevalLibrary_dGetMultipleComputationsResults(void* handle_pacevalComputations_in[],
unsigned long numberOfpacevalComputations_in, double values_in[],
double* results_out, double* trustedMinResults_out, double* trustedMaxResults_out,
int* errorTypes_out);
bool pacevalLibrary_fGetMultipleComputationsResults(void* handle_pacevalComputations_in[],
unsigned long numberOfpacevalComputations_in, float values_in[],
float* results_out, float* trustedMinResults_out, float* trustedMaxResults_out,
int* errorTypes_out);
bool pacevalLibrary_ldGetMultipleComputationsResults(void* handle_pacevalComputations_in[],
unsigned long numberOfpacevalComputations_in, long double values_in[],
long double* results_out, long double* trustedMinResults_out, long double* trustedMaxResults_out,
int* errorTypes_out);
Please, read Foreign function interface for more information and examples.
EXAMPLE
see examples “paceval_example4.cpp” or “paceval_example6.cpp” of the paceval. Software Development Kit
//This code creates two mathematical functions in parallel and then also performs two calculations in parallel.
//Of course the effort for these simple functions doesn’t make sense :), but imagine 10 or more functions,
//each with a length of hundreds or millions of characters and with 10 or more variables.
//In this case, the performance, readability and maintainability of paceval. will come into play.
//For an example with interval arithmetic, just look here: https://paceval.com/simple-code-example/
PACEVAL_HANDLE* handle_pacevalComputationsArray = new PACEVAL_HANDLE[2];
const char** functionStringsArray = new const char*[2];
char* functionString_Addition = new char[sizeof(“5+x”) + 1];
char* functionString_Multiplication = new char[sizeof(“y*7.8”) + 1];
double* results = new double[2];
double valuesVariablesArray[2];
int* errorTypes = new int[2];
bool hasError;
strcpy(functionString_Addition, “5+x”);
strcpy(functionString_Multiplication, “y*7.8”);
functionStringsArray[0] = functionString_Addition;
functionStringsArray[1] = functionString_Multiplication;
hasError = paceval_CreateMultipleComputations(handle_pacevalComputationsArray, functionStringsArray, 2, 2, “x y”, false, NULL);
delete[] functionString_Addition;
delete[] functionString_Multiplication;
delete[] functionStringsArray;
valuesVariablesArray[0] = 2.6; //value for variable x
valuesVariablesArray[1] = -5; //value for variable y
hasError = paceval_dGetMultipleComputationsResults(handle_pacevalComputationsArray, 2, valuesVariablesArray, &results[0], NULL, NULL, &errorTypes[0]);
//results[0] will be 7.6 (5+2.6)
//results[1] will be -39 (-5*7.8)
paceval_DeleteComputation(handle_pacevalComputationsArray[0]);
paceval_DeleteComputation(handle_pacevalComputationsArray[1]);
delete[] handle_pacevalComputationsArray;
delete[] errorTypes;
delete[] results;
bool paceval_dGetMultipleComputationsResultsExt(
PACEVAL_HANDLE handle_pacevalComputations_in[],
unsigned long numberOfpacevalComputations_in,
double values_in[],
unsigned long numberOfCalculations_in,
double* results_out,
double* trustedMinResults_out,
double* trustedMaxResults_out,
int* errorTypes_out);
use paceval_fGetMultipleComputationsResultsExt(…) for float values
use paceval_ldGetMultipleComputationsResultsExt(…) for long double values
NAME
paceval_dGetMultipleComputationsResultsExt
paceval_fGetMultipleComputationsResultsExt
paceval_ldGetMultipleComputationsResultsExt
DESCRIPTION
Performs multiple calculations in parallel on multiple paceval.-Computation objects using the variables declared by paceval_CreateComputation() and with multiple sets of values for those variables, i.e. in mathematical notation
You should use paceval_dGetMultipleComputationsResultsExt() to get results for multiple computations at the same time and to carry out as many different evaluations as possible at once (e.g. for artificial neural network functions or decision trees in which several functions with different variable values are calculated in one step for several objects at the same time to decide).
Annotation: You must use the same number of variables and the same declared variables. To do so you can simply declare all variables in each of the the calls for paceval_CreateComputation(). There is no performance issue in case a variable is declared but not used by a paceval.-Computation object.
PARAMETERS
PACEVAL_HANDLE handle_pacevalComputation_in[] – Array of the paceval.-Computation objects.
unsigned long numberOfpacevalComputations_in – Specifies the number of the paceval.-Computation objects.
double values_in[] – Array of double defining the values of the variables.
unsigned long numberOfCalculations_in – Specifies the number of different calculations you want to run. This number specifies the size of the array values_in[]. E.g. if the number of variables declared is 50 and the number of calculations is 1000 the size of the array values_in[] must be 50*1000. The order of the array values_in[] must be variable values for the first calculation, then variable values for the second calculation and so on.
double* results_out – Pointer to an array for the results of the multiple computations.
Annotation: In case of an error the values are not specified.
double* trustedMinResult_out – Pointer to an array for the minimum/lower interval limits.
Annotation: In case of an error the values are not specified.
double* trustedMaxResult_out – Pointer to an array for the maximum/upper interval limits.
Annotation: In case of an error the value is not specified.
int* errorTypes_out – Pointer to an array of the paceval.-Computation object errors, see also paceval_eErrorTypes in paceval_main.h or paceval_GetErrorInformation().
RETURN VALUE
bool – The return value indicates paceval.-Computation object errors. You should check the array errorTypes_out in this case.
FOREIGN FUNCTION INTERFACE
bool pacevalLibrary_dGetMultipleComputationsResultsExt(void* handle_pacevalComputations_in[],
unsigned long numberOfpacevalComputations_in, double values_in[],
unsigned long numberOfCalculations_in, double* results_out,
double* trustedMinResults_out, double* trustedMaxResults_out,
int* errorTypes_out);
bool pacevalLibrary_fGetMultipleComputationsResultsExt(void* handle_pacevalComputations_in[],
unsigned long numberOfpacevalComputations_in, float values_in[],
unsigned long numberOfCalculations_in, float* results_out,
float* trustedMinResults_out, float* trustedMaxResults_out,
int* errorTypes_out);
bool pacevalLibrary_ldGetMultipleComputationsResultsExt(void* handle_pacevalComputations_in[],
unsigned long numberOfpacevalComputations_in, long double values_in[],
unsigned long numberOfCalculations_in, long double* results_out,
long double* trustedMinResults_out, long double* trustedMaxResults_out,
int* errorTypes_out);
Please, read Foreign function interface for more information and examples.
EXAMPLE C/C++
//This code creates two mathematical functions in parallel and then performs calculations in parallel
//with three different sets of values for the variables.
//Of course the effort for these simple functions and three sets for the variables doesn’t make sense :), but
//imagine 10 or more functions, each with a length of hundreds or millions of characters and with 10 or more
//variables and 100 or more sets for the variables.
//In this case, the performance, readability and maintainability of paceval will come into play.
//For an example with interval arithmetic, just look here: https://paceval.com/simple-code-example/
PACEVAL_HANDLE* handle_pacevalComputationsArray = new PACEVAL_HANDLE[2];
const char** functionStringsArray = new const char*[2];
char* functionString_Addition = new char[sizeof(“5+x”) + 1];
char* functionString_Multiplication = new char[sizeof(“y*7.8”) + 1];
double* results = new double[6];
double valuesVariablesArray[6];
int* errorTypes = new int[6];
bool hasError;
strcpy(functionString_Addition, “5+x”);
strcpy(functionString_Multiplication, “y*7.8”);
functionStringsArray[0] = functionString_Addition;
functionStringsArray[1] = functionString_Multiplication;
hasError = paceval_CreateMultipleComputations(handle_pacevalComputationsArray, functionStringsArray, 2, 2, “x y”, false, NULL);
delete[] functionString_Addition;
delete[] functionString_Multiplication;
delete[] functionStringsArray;
valuesVariablesArray[0] = 2.6; //first value for variable x
valuesVariablesArray[1] = 5.8; //first value for variable y
valuesVariablesArray[2] = -1.2; //second value for variable x
valuesVariablesArray[3] = 6.9; //second value for variable y
valuesVariablesArray[4] = -1.8; //third value for variable x
valuesVariablesArray[5] = 12.1; //third value for variable y
hasError = paceval_dGetMultipleComputationsResultsExt(handle_pacevalComputationsArray, 2, valuesVariablesArray, 3, &results[0], NULL, NULL, &errorTypes[0]);
//results[0] will be 7.6 (5+2.6)
//results[1] will be 3.8 (5-1.2)
//results[2] will be 3.2 (5-1.8)
//results[3] will be 45,24 (5.8*7.8)
//results[4] will be 53,82 (6.9*7.8)
//results[5] will be 94,38 (12.1*7.8)
paceval_DeleteComputation(handle_pacevalComputationsArray[0]);
paceval_DeleteComputation(handle_pacevalComputationsArray[1]);
delete[] handle_pacevalComputationsArray;
delete[] errorTypes;
delete[] results;
double paceval_dmathv(
PACEVAL_HANDLE* handle_pacevalComputation_out_in,
int* errorType_out,
const char* functionString_in,
unsigned long numberOfVariables_in,
const char* variables_in,
…);
use paceval_fmathv(…) for float values
use paceval_ldmathv(…) for long double values
NAME
paceval_dmathv
paceval_fmathv
paceval_ldmathv
DESCRIPTION
A convenience function to get results of computations in a single step.
PARAMETERS
PACEVAL_HANDLE* handle_pacevalComputation_out_in – Pointer to a paceval.-Computation object. If handle_pacevalComputation_out_in is not NULL this buffer will retrieve the created paceval.-Computation object and can be used again. This will improve the performance. In this case the paceval.-Computation object will not be deleted and you must call paceval_DeleteComputation().
int* errorType_out – Points to the buffer that will receive the paceval.-Computation object error, see also paceval_eErrorTypes in paceval_main.h or paceval_GetErrorInformation().
const char* functionString_in – Points to a null-terminated string to be used as the function to work with.
see also Product brief-Supported terms
unsigned long numberOfVariables_in – Specifies the number of variables to work with. E.g. if the variables are “xValue yValue zValue” or “x y z” numberOfVariables_in must be set to 3.
const char* variables_in – Points to a null-terminated string specifying the names of the working variables. Generally the variables must be separated by a blank. E.g. the variables xValue, yValue, zValue must be set with “xValue yValue zValue” or the variables x, y, z must be set with “x y z”.
… – Parameters comma seperated will be interpreted as double values for the variables.
RETURN VALUE
double – If the function succeeds, the return value is the result of the computation.
paceval_GetIsError() should be called to check whether an error has occurred. To get more information about the error, call paceval_GetErrorInformation().
Annotation: In case of an error the return value is not specified.
FOREIGN FUNCTION INTERFACE
double pacevalLibrary_dmathv(void* handle_pacevalComputation_out_in,
int* errorType_out, const char* functionString_in,
unsigned long numberOfVariables_in, const char* variables_in, double values_in[]);
float pacevalLibrary_fmathv(void* handle_pacevalComputation_out_in,
int* errorType_out, const char* functionString_in,
unsigned long numberOfVariables_in, const char* variables_in, float values_in[]);
long double pacevalLibrary_ldmathv(void* handle_pacevalComputation_out_in,
int* errorType_out, const char* functionString_in,
unsigned long numberOfVariables_in, const char* variables_in, long double values_in[]);
Please, read Foreign function interface for more information and examples.
EXAMPLE C/C++
double result;
int errType;
result = paceval_dmathv(NULL, &errType, “5*6^(12/3)“, 0, “”, NULL);
//result will be 6480
result = paceval_dmathv(NULL, &errType, “-sin(x*cos(x))^(1/y)”, 2, “x y”, 0.5, 2.0);
//result will be -0.651801782452278
double paceval_dmathvi(
PACEVAL_HANDLE* handle_pacevalComputation_out_in,
int* errorType_out,
double* trustedMinResult_out,
double* trustedMaxResult_out,
const char* functionString_in,
unsigned long numberOfVariables_in,
const char* variables_in,
…);
use paceval_fmathvi(…) for float values
use paceval_ldmathvi(…) for long double values
NAME
paceval_dmathvi
paceval_fmathvi
paceval_ldmathvi
DESCRIPTION
A convenience function to get results of computations in a single step with Trusted Interval Computation, TINC (paceval. specific).
PARAMETERS
PACEVAL_HANDLE* handle_pacevalComputation_out_in – Pointer to a paceval.-Computation object. If handle_pacevalComputation_in is not NULL this buffer will retrieve the created paceval.-Computation object and can be used again. This will improve the performance. In this case the paceval.-Computation object will not be deleted and you must call paceval_DeleteComputation().
int* errorType_out – Points to the buffer that will receive the paceval.-Computation object error, see also paceval_eErrorTypes in paceval_main.h or paceval_GetErrorInformation().
double* trustedMinResult_out – This buffer retrieves the minimum/lower interval limit of the computation.
Annotation: In case of an error the value is not specified.
double* trustedMaxResult_out – This buffer retrieves the maximum/upper interval limit of the computation.
Annotation: In case of an error the value is not specified.
const char* functionString_in – Points to a null-terminated string to be used as the function to work with.
see also Product brief-Supported terms
unsigned long numberOfVariables_in – Specifies the number of variables to work with. E.g. if the variables are “xValue yValue zValue” or “x y z” numberOfVariables_in must be set to 3.
const char* variables_in – Points to a null-terminated string specifying the names of the working variables. Generally the variables must be separated by a blank. E.g. the variables xValue, yValue, zValue must be set with “xValue yValue zValue” or the variables x, y, z must be set with “x y z”.
… – Parameters comma seperated will be interpreted as double values for the variables.
RETURN VALUE
double – If the function succeeds, the return value is the result of the computation.
paceval_GetIsError() should be called to check whether an error has occurred. To get more information about the error, call paceval_GetErrorInformation().
Annotation: In case of an error the return value is not specified.
FOREIGN FUNCTION INTERFACE
double pacevalLibrary_dmathvi(void* handle_pacevalComputation_out_in,
int* errorType_out, double* trustedMinResult_out, double* trustedMaxResult_out,
const char* functionString_in, unsigned long numberOfVariables_in,
const char* variables_in, double values_in[]);
float pacevalLibrary_fmathvi(void* handle_pacevalComputation_out_in,
int* errorType_out, float* trustedMinResult_out, float* trustedMaxResult_out,
const char* functionString_in, unsigned long numberOfVariables_in,
const char* variables_in, float values_in[]);
long double pacevalLibrary_ldmathvi(void* handle_pacevalComputation_out_in,
int* errorType_out, long double* trustedMinResult_out, long double* trustedMaxResult_out,
const char* functionString_in, unsigned long numberOfVariables_in,
const char* variables_in, long double values_in[]);
Please, read Foreign function interface for more information and examples.
EXAMPLE C/C++
double result, minResultInterval, maxResultInterval;
int errType;
result = paceval_dmathvi(NULL, &errType, &minResultInterval, &maxResultInterval, “-sin(x*cos(x))^(1/y)”, 2, “x y”, 0.5, 2.0);
//result will be -0.651801782452278, minResultInterval will be -0.651801782452306, maxResultInterval will be -0.65180178245225
unsigned long paceval_CreateXMLFromParameters(
char* paceval_strXML_out,
const char* functionString_in,
unsigned long numberOfVariables_in,
const char* variables_in,
const char* valuesString_in,
bool useInterval_in);
NAME
paceval_CreateXMLFromParameters
DESCRIPTION
A helper function to create XML data representing a paceval.-Computation object. You can use it to store data representing a computation to a file system or to transmit it via a network or channel.
PARAMETERS
char* paceval_strXML_out – Pointer to a buffer for the XML data. Set paceval_strXML_out to NULL to receive the length of the version string, see RETURN VALUE.
const char* functionString_in – Points to a null-terminated string representing the function.
see also Product brief-Supported terms
unsigned long numberOfVariables_in – Specifies the number of variables.
const char* variables_in – Points to a null-terminated string specifying the names of the variables in one string seperated by blanks.
const char* valuesString_in – Specifies the values for the variables in one string seperated by semicolons (;).
bool useInterval_in – Specifies whether Trusted Interval Computation is enabled or not.
RETURN VALUE
unsigned long – The return value is the size of the XML data. In case of an error the return value is 0.
FOREIGN FUNCTION INTERFACE
unsigned long pacevalLibrary_CreateXMLFromParameters(char* paceval_strXML_out,
const char* functionString_in, unsigned long numberOfVariables_in,
const char* variables_in, const char* valuesString_in, bool useInterval_in);
Please, read Foreign function interface for more information and examples.
EXAMPLE
see demo application AppCalculation “main.cpp” of the paceval. Software Development Kit
see example “paceval_example5.cpp” of the paceval. Software Development Kit
int paceval_ReadParametersFromXML(
const char* paceval_strXML_in,
unsigned long* functionStringLength_out,
unsigned long* variablesStringLength_out,
unsigned long* numberOfVariables_out,
unsigned long* valuesStringLength_out,
char* functionString_out,
char* variables_out,
char* valuesString_out,
bool* useInterval_out);
NAME
paceval_ReadParametersFromXML
DESCRIPTION
A helper function to read XML data representing a paceval.-Computation object. You can use it to read data representing a computation from a file system or to receive it via a network or channel.
PARAMETERS
char* paceval_strXML_in – Pointer to the XML data.
unsigned long* functionStringLength_out – The length of the function string.
unsigned long* variablesStringLength_out – The length of the variable names in one string.
unsigned long* numberOfVariables_out – The number of variables.
unsigned long* valuesStringLength_out – The length of the values seperated by blanks in one string.
char* functionString_out – Points to the buffer for the function string. Set functionString_out to NULL to receive the length at first with functionStringLength_out.
char* variables_out – Points to the buffer for the variable names in one string. Set variables_out to NULL to receive the length at first with variablesStringLength_out.
char* valuesString_out – Points to the buffer for the values seperated by semicolons (;) in one string. Set valuesString_out to NULL to receive the length at first with valuesStringLength_out.
bool* useInterval_out – Points to the buffer specifying whether Trusted Interval Computation is enabled or not.
RETURN VALUE
int – In case of an error the return value is <0.
FOREIGN FUNCTION INTERFACE
int pacevalLibrary_ReadParametersFromXML(const char* paceval_strXML_in,
unsigned long* functionStringLength_out, unsigned long* variablesStringMaximumLength_out,
unsigned long* numberOfVariables_out, unsigned long* valuesStringMaximumLength_out,
char* functionString_out, char* variables_out, char* valuesString_out, bool* useInterval_out);
Please, read Foreign function interface for more information and examples.
EXAMPLE
see demo application AppCalculation “main.cpp” of the paceval. Software Development Kit
see example “paceval_example5.cpp” of the paceval. Software Development Kit
unsigned long paceval_GetComputationInformationXML(
PACEVAL_HANDLE* handle_pacevalComputation_in,
char* paceval_strXML_out);
NAME
paceval_GetComputationInformationXML
DESCRIPTION
A helper function for getting data from a paceval.-Computation object, such as the number of cores in the system, the number of threads used or the cache hits in the computations with that particular paceval.-Computation object.
PARAMETERS
PACEVAL_HANDLE handle_pacevalComputation_in – Identifies the paceval.-Computation object.
char* paceval_strXML_out – Pointer to a buffer for the XML data. Set paceval_strXML_out to NULL to receive the length of the version string, see RETURN VALUE.
RETURN VALUE
unsigned long – The return value is the size of the XML data. In case of an error the return value is 0.
FOREIGN FUNCTION INTERFACE
unsigned long paceval_GetComputationInformationXML(void* handle_pacevalComputation_in,
char* paceval_strXML_out);
Please, read Foreign function interface for more information and examples.
EXAMPLE
This is an example for the generated XML data:
<?xml version=”1.0″ encoding=”ISO-8859-1″ standalone=”yes”?>
<paceval.-Computation>
<version>4.01</version>
<function50Characters>isnull(x-(0))*(0) + ispos(x-(0))*isposq((0.025)-x)(…)</function50Characters>
<functionLength>28630</functionLength>
<numberOfVariables>1000</numberOfVariables>
<useInterval>false</useInterval>
<errorMessage>No error occured for this computation object (PACEVAL_ERR_NO_ERROR).</errorMessage>
<errorDetails>[NO ERROR]</errorDetails>
<maxPrecisionType>long double</maxPrecisionType>
<numberOfNodes>6888</numberOfNodes>
<numberOfCores>16</numberOfCores>
<numberOfThreads>10</numberOfThreads>
<cacheTypes>Lookahead Caching</cacheTypes>
<cacheHitsACC>132</cacheHitsACC>
</paceval.-Computation>
int paceval_CreateErrorInformationText(
PACEVAL_HANDLE handle_pacevalComputation_in,
char* lastError_strMessage_out,
char* lastError_strDetails_out);
NAME
paceval_CreateErrorInformationText
DESCRIPTION
The paceval_CreateErrorInformationText() is a helper function to create error information in text from a paceval.-Computation object. It can be used to provide more information to the user like in the screenshot.
For more details see paceval_GetIsError() and paceval_GetErrorInformation().
PARAMETERS
PACEVAL_HANDLE handle_pacevalComputation_in – Identifies the paceval.-Computation object.
char* lastError_strMessage_out – Buffer to get message describing the error.
Annotation: Maximum size of the retrieved null-terminated string is 255 per “#define PACEVAL_MAXERR 255”, see paceval_main.h.
char* lastError_strDetails_out – Buffer to get details like error type as a number, the operator and the position in the function.
Annotation: Maximum size of the retrieved null-terminated string is 255 per “#define PACEVAL_MAXERR 255”, see paceval_main.h.
RETURN VALUE
int – The return value is maximum length of lastError_strMessage_out and lastError_strDetails_out.
FOREIGN FUNCTION INTERFACE
int pacevalLibrary_CreateErrorInformationText(void* handle_pacevalComputation_in,
char* lastError_strMessage_out, char* lastError_strDetails_out);
Please, read Foreign function interface for more information and examples.
EXAMPLE
see demo application AppCalculation “main.cpp” of the paceval. Software Development Kit
see examples “paceval_example1.cpp” or “paceval_example5.cpp” of the paceval. Software Development Kit
int paceval_GetErrorTypeMessage(
int errorType_in,
char* lastError_strMessage_out);
NAME
paceval_GetErrorTypeMessage
DESCRIPTION
The paceval_GetErrorTypeMessage() is a helper function to create an error message in text from an error type. It can be used to provide more information to the user like in the screenshot.
For more details see paceval_GetIsError() and paceval_GetErrorInformation().
PARAMETERS
int errorType_in – Specifies the the error type value.
char* lastError_strMessage_out – Buffer to get message describing the error.
Annotation: Maximum size of the retrieved null-terminated string is 255 per “#define PACEVAL_MAXERR 255”, see paceval_main.h.
RETURN VALUE
int – The return value is the length of lastError_strMessage_out.
FOREIGN FUNCTION INTERFACE
int pacevalLibrary_GetErrorTypeMessage(int errorType_in, char* errorType_strMessage_out);
Please, read Foreign function interface for more information and examples.
EXAMPLE
see demo application AppCalculation “main.cpp” or “graph.cpp” of the paceval. Software Development Kit
see examples “paceval_example2.cpp”, “paceval_example3.cpp”, “paceval_example4.cpp” or “paceval_example6.cpp” of the paceval. Software Development Kit
bool paceval_SetCallbackUserFunction(
unsigned int numberUserFunction_in,
const char* singleFunctionString_in,
paceval_callbackUserFunctionType* paceval_callbackUserFunction_in);
NAME
paceval_SetCallbackUserFunction
DESCRIPTION
Allows the definition of up to 1000 custom user functions. Depending on your requirements, you could
a) add mathematical functions that enable new functions, e.g. with Boost
or
b) add faster mathematical functions that are not as accurate as those used by paceval., e.g. math-neon.
PARAMETERS
unsigned int numberUserFunction_in – Specifies the number of the user function to set the single function for, e.g. 1.
const char* singleFunctionString_in – Points to a null-terminated string specifying the user-defined single function, e.g. “my_function1”.
paceval_callbackUserFunctionType* paceval_callbackUserFunction_in – A user-defined callback function to handle the computation for the specific single function, see paceval_main.h:
typedef bool paceval_callbackUserFunctionType(const int, const long double*, long double*,
const double*, double*, const float*, float*);
RETURN VALUE
bool – If the function succeeds, the return value is true. Otherwise it is false.
FOREIGN FUNCTION INTERFACE
bool pacevalLibrary_SetCallbackUserFunction(unsigned int numberUserFunction_in,
const char* singleFunctionString_in, paceval_callbackUserFunctionType* paceval_callbackUserFunction_in);
Please, read Foreign function interface for more information and examples.
EXAMPLE
see demo application AppCalculation “main.cpp” of the paceval. Software Development Kit
see example “paceval_example2.cpp” of the paceval. Software Development Kit
EXAMPLE C/C++ and Boost
#include <boost/math/special_functions.hpp> // for Gamma function
bool paceval_callbackUserFunctionGamma(const int useCalculationPrecision_in,
const long double* valueFieldAsLongDouble, long double* resultAsLongDouble,
const double* valueFieldAsDouble, double* resultAsDouble,
const float* valueFieldAsFloat, float* resultAsFloat)
{
switch (useCalculationPrecision_in)
{
case PACEVAL_CALCULATION_PRECISION_LONG_DOUBLE:
{
*resultAsLongDouble = tgamma<long double>(*valueFieldAsLongDouble);
return true;
}
case PACEVAL_CALCULATION_PRECISION_DOUBLE:
{
*resultAsDouble = tgamma<double>(*valueFieldAsDouble);
return true;
}
case PACEVAL_CALCULATION_PRECISION_FLOAT:
{
*resultAsFloat = = tgamma<float>(*valueFieldAsFloat);
return true;
}
}
return false;
}
int main(int argc, char* argv[])
{
paceval_InitializeLibrary(NULL);
bool success = paceval_SetCallbackUserFunction(1, “boost_gamma”,
paceval_callbackUserFunctionGamma);
… //your code here
return 0;
}
How to easily call paceval.-library functions from another programming language
Background and examples : Foreign function interface (FFI)
A foreign function interface (FFI) is a mechanism by which a program written in one programming language can call routines or make use of services written in another, see https://en.wikipedia.org/wiki/Foreign_function_interface. Since paceval. is written in C/C++, it is very easy to use functions from a paceval.-library in a lot of other programming languages like .NET, ABAP, C, C#, COBOL, Delphi, Erlang, Fortran, Golang, Java, Maple, Julia, Kotlin, Lua, Mathematica, MATLAB, Mendix, Node.js, Octave, Pascal, Perl, PHP, Python, R, Ruby, Rust, Scratch, Swift, TypeScript or Visual Basic (just follow the links for the explanations regarding FFI for the different programming languages).
We have created Docker images for paceval-services running on Linux servers for x86 processors (Intel and AMD) and ARM64 to perform mathematical calculations on a remote computer or server.
The setup instructions can be found here: paceval-service-a Linux server for x64 processors (Intel and AMD).pdf
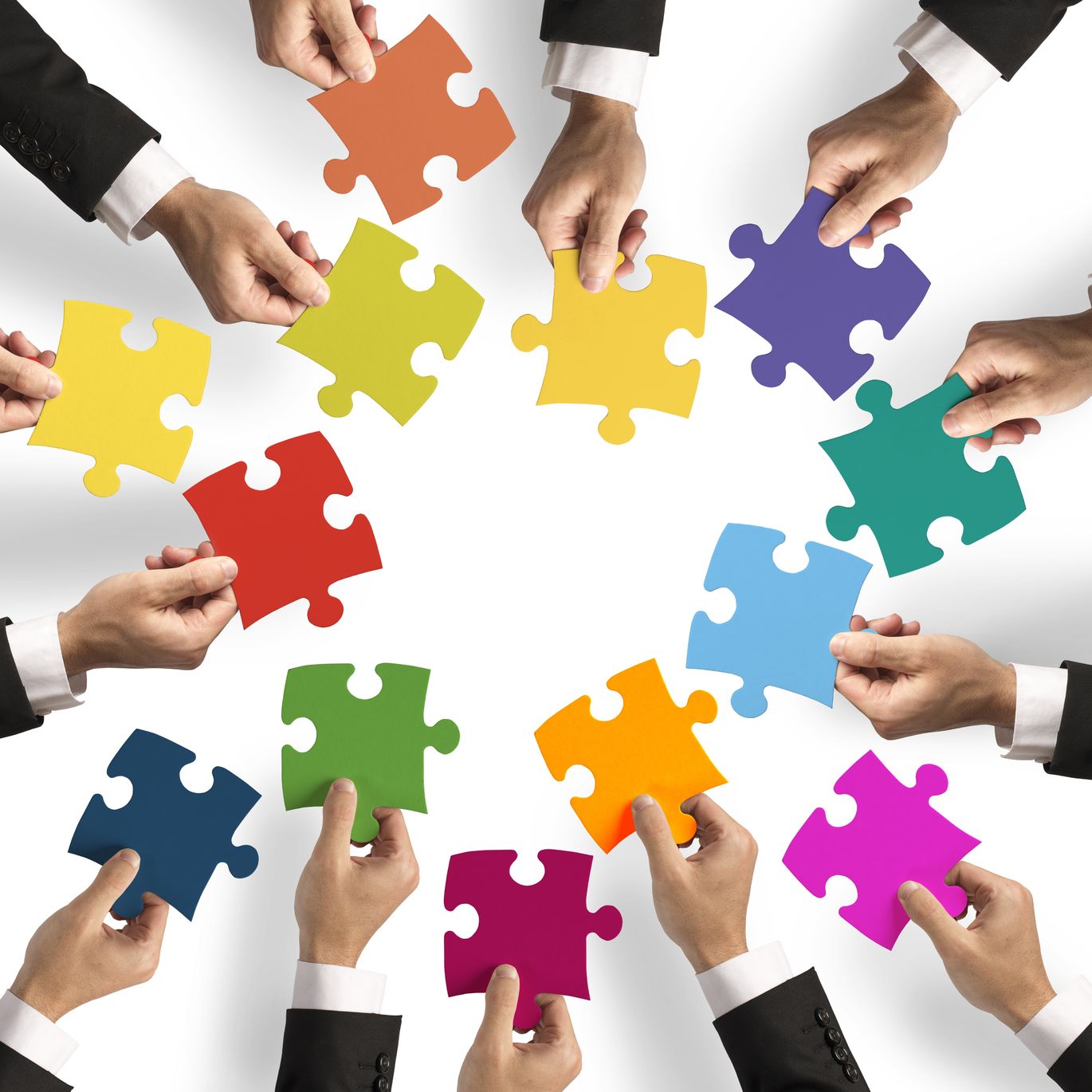
The general approach for all programming languages is usually pretty much the same, so we will use PHP in our examples below. Since PHP 7.4 it is possible to call C functions from shared libraries (.dll or .so). You may need to change the php.ini to enable FFI.
You can use the following examples to build your own powerful RESTful API.
Or you can create your own mathematical engine in the cloud, e.g. to offload battery-operated IoT devices from battery-consuming calculations, see example mathematical-engine and source-code below (see https://app.swaggerhub.com/apis-docs/paceval/paceval-service/4.04 for more details). You can also run cUrl instructions with your command line or your IoT device directly on our test server (paceval-service.com), like the following:
Note: Use the correct encoding in the functionString in the URL (GET) and data (POST) (e.g. replace the ‘+’ character with ‘%2B’).
GET –
curl -X GET -k "http://paceval-service.com:80/Demo/?functionString=-sin(x*cos(x))^(1/y)&numberOfVariables=2&variables=x;y&values=0.5;2&interval=yes"
or POST –
curl --data "functionString=-sin(x*cos(x))^(1/y)&numberOfVariables=2&variables=x;y&values=0.5;2&interval=yes" -X POST http://paceval-service.com:80/Demo/
Links & Literature
[1] https://en.wikipedia.org/wiki/Foreign_function_interface
[2] https://wiki.php.net/rfc/ffi
[3] https://docs.python.org/3/library/ctypes.html
[4] https://en.wikipedia.org/wiki/JSON
[5] https://en.wikipedia.org/wiki/CURL
[6] Just follow the links above for the explanations regarding FFI for the different programming languages.
EXAMPLES – PHP